
admin 패키지에 common 패키지 생성후 안에 Constants.java 생성 |
->Constants.java 파일은 서비스내의 공통변수를 모아넣은 클래스 파일임
package com.board.common;
/** 서비스에 사용되는 공통변수 */
public final class Constants {
//프로젝트 패키지 이름
public final static String APP_DEFAULT_PACKAGE_NAME = "com.board";
//dao 패키지 경로
public final static String MAPPER_PACKAGE = Constants.APP_DEFAULT_PACKAGE_NAME+".dao";
//Tiles xml 경로
public final static String[] TILES_LAYOUT_XML_PATH = {
"WEB-INF/tiles.xml"
};
//Runtime에서 JSP의 refresh 적용 여부
public final static boolean REFRESH_JSP_ON_RUNTIME = true;
}
전장에 만들었던 Admin 프로젝트 run 파일 수정 (AdminApplication.java 수정) |
AdminApplication.java |
package com.board.admin;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.ComponentScan;
import com.board.common.Constants;
@SpringBootApplication
@ComponentScan(basePackages = Constants.APP_DEFAULT_PACKAGE_NAME)
@MapperScan(basePackages = Constants.MAPPER_PACKAGE)
public class AdminApplication {
public static void main(String[] args) {
SpringApplication.run(AdminApplication.class, args);
}
}
-> 공통변수로 바꿔줌
admin 프로젝트 config 패키지 생성후 안에 TilesConfig.java 생성 |
TilesConfig.java |
package com.board.config;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.view.tiles3.TilesConfigurer;
import org.springframework.web.servlet.view.tiles3.TilesView;
import org.springframework.web.servlet.view.tiles3.TilesViewResolver;
import com.board.common.Constants;
@Configuration
public class TilesConfig {
@Bean
public TilesConfigurer tilesConfigurer() {
final TilesConfigurer configurer = new TilesConfigurer();
configurer.setDefinitions(Constants.TILES_LAYOUT_XML_PATH);
configurer.setCheckRefresh(true);
return configurer;
}
@Bean
public TilesViewResolver tilesViewResolver() {
final TilesViewResolver tilesViewResolver = new TilesViewResolver();
tilesViewResolver.setViewClass(TilesView.class);
return tilesViewResolver;
}
}
main 폴더아래 webapp, WEB-INF 폴더 생성후 WEB-INF 폴더안에 template, views 폴더 생성 |
admin 프로젝트 WEB-INF 폴더 밑에 tiles.xml 생성 |
tiles.xml |
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE tiles-definitions PUBLIC "-//Apache Software Foundation//DTD Tiles Configuration 3.0//EN" "http://tiles.apache.org/dtds/tiles-config_3_0.dtd">
<tiles-definitions>
<!-- 레이아웃 템플릿 정의 -->
<definition name="tiles/default/template" template="/WEB-INF/template/template.jsp">
<put-attribute name="meta" value="/WEB-INF/template/common/meta.jsp"/>
<put-attribute name="styles" value="/WEB-INF/template/common/styles.jsp"/>
<put-attribute name="scripts" value="/WEB-INF/template/common/scripts.jsp"/>
<put-attribute name="header" value="/WEB-INF/template/common/header.jsp"/>
<put-attribute name="snb" value="/WEB-INF/template/common/snb.jsp"/>
</definition>
<!-- Depth(4) : / A / B / C / D / E.jsp -->
<definition name="/tiles/view/*/*/*/*/*" extends="tiles/default/template">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}/{3}/{4}/{5}.jsp"/>
<put-attribute name="contents-js" value="/WEB-INF/views/{1}/{2}/{3}/{4}/{5}-js.jsp"/>
</definition>
<!-- Depth(3) : / A / B / C / D.jsp -->
<definition name="/tiles/view/*/*/*/*" extends="tiles/default/template">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}/{3}/{4}.jsp"/>
<put-attribute name="contents-js" value="/WEB-INF/views/{1}/{2}/{3}/{4}-js.jsp"/>
</definition>
<!-- Depth(2) : / A / B / C.jsp -->
<definition name="/tiles/view/*/*/*" extends="tiles/default/template">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}/{3}.jsp"/>
<put-attribute name="contents-js" value="/WEB-INF/views/{1}/{2}/{3}-js.jsp"/>
</definition>
<!-- Depth(1) : / A / B.jsp -->
<definition name="/tiles/view/*/*" extends="tiles/default/template">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}.jsp"/>
<put-attribute name="contents-js" value="/WEB-INF/views/{1}/{2}-js.jsp"/>
</definition>
<!-- Depth(0) : / A.jsp -->
<definition name="/tiles/view/*" extends="tiles/default/template">
<put-attribute name="contents" value="/WEB-INF/views/{1}.jsp"/>
<put-attribute name="contents-js" value="/WEB-INF/views/{1}-js.jsp"/>
</definition>
<!-- 화면 영역 분할 등에서 사용함 -->
<definition name="tiles/default/template-simple" template="/WEB-INF/template/template-simple.jsp">
</definition>
<!-- Depth(4) : / A / B / C / D / E.jsp -->
<definition name="/tiles/ajax/*/*/*/*/*" extends="tiles/default/template-simple">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}/{3}/{4}/{5}.jsp"/>
</definition>
<!-- Depth(3) : / A / B / C / D.jsp -->
<definition name="/tiles/ajax/*/*/*/*" extends="tiles/default/template-simple">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}/{3}/{4}.jsp"/>
</definition>
<!-- Depth(2) : / A / B / C.jsp -->
<definition name="/tiles/ajax/*/*/*" extends="tiles/default/template-simple">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}/{3}.jsp"/>
</definition>
<!-- Depth(1) : / A / B.jsp -->
<definition name="/tiles/ajax/*/*" extends="tiles/default/template-simple">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}.jsp"/>
</definition>
<!-- Depth(0) : / A.jsp -->
<definition name="/tiles/ajax/*" extends="tiles/default/template-simple">
<put-attribute name="contents" value="/WEB-INF/views/{1}.jsp"/>
</definition>
<!-- 에러 페이지 레이아웃 -->
<definition name="tiles/error/template" template="/WEB-INF/template/error/error-template.jsp"/>
<definition name="/errors/*" extends="tiles/error/template">
<put-attribute name="contents" value="/WEB-INF/errors/{1}.jsp"/>
</definition>
</tiles-definitions>
-> tiles를 크게 meta, styles, scripts, header, snb, template로 나눔
- meta는 메타 태그 관련된 코드가 들어가는 부분
- styles는 css 파일들을 import 할수 있는 코드들이 들어가 있는 부분
- scripts는 js 파일들을 import 할수 있는 코드들이 들어가 있는 부분
- snb는 snb 코드가 들어가는 부분
- template는 <tiles:insertAttribute>를 사용해서 meta, styles, scripts, content, content-js 영역등을 설정해서 화면에 나타냄
template 폴더안에 common 폴더 생성 |
-> common폴더안에 header, meta, scripts, snb, styles, template, template-simple 생성
template 폴더안에 error 폴더 생성 |
- error 폴더안에 error-template.jsp 생성
error-template.jsp |
<%@ page contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
<%@ taglib prefix="tiles" uri="http://tiles.apache.org/tags-tiles" %>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
</head>
<body>
<tiles:insertAttribute name="contents"/>
</body>
</html>
-> 일단 위에꺼만 만들어놓고 게시판을 만들면서 header, styles, scripts, snb등은 추후에 만들예정임
template폴더안에 template.jsp, template-simple.jsp, constants.jsp 생성 |
template.jsp |
<%@ page contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
<%@ taglib prefix="tiles" uri="http://tiles.apache.org/tags-tiles" %>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
</head>
<body>
<tiles:insertAttribute name="contents"/>
<tiles:insertAttribute name="contents-js"/>
</body>
</html>
template-simple.jsp 생성 |
<%@ page contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
<%@ taglib prefix="tiles" uri="http://tiles.apache.org/tags-tiles" %>
<!DOCTYPE html>
<html lang="ko">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
</head>
<body>
<tiles:insertAttribute name="contents"/>
</body>
</html>
constants.jsp |
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<%@ taglib prefix="fn" uri="http://java.sun.com/jsp/jstl/functions" %>
<%@ taglib prefix="spring" uri="http://www.springframework.org/tags" %>
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form" %>
<c:set var="version" value="<%=new java.util.Date()%>" />
<c:set var="ctxPath" value="${pageContext.request.contextPath eq '/' ? '' : pageContext.request.contextPath}" />
<c:set var="remoteURI" value="${ctxPath}${requestScope['javax.servlet.forward.servlet_path']}" />
<c:set var="orgRemoteURI" value="${requestScope['javax.servlet.forward.servlet_path']}" />
<sec:authentication property="principal" var="principal" />
-> jstl등 태그 라이브러리 import 코드가 모여있는 jsp파일임
WEB-INF/views/auth 폴더안에 해당 파일 만들기 |
login.jsp (로그인화면) |
<%@ page language="java" session="true" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%>
<%@ include file="/WEB-INF/template/constants.jsp"%>
<link href="//maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" rel="stylesheet" id="bootstrap-css">
<script src="//maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script>
<script src="//cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<!------ Include the above in your HEAD tag ---------->
<link rel="stylesheet" type="text/css" href="${ctxPath}/css/loginForm.css"/>
<div class="wrapper fadeInDown">
<div id="formContent">
<!-- Tabs Titles -->
<!-- Icon -->
<div class="fadeIn first">
로그인
</div>
<!-- Login Form -->
<form>
<input type="text" id="userId" class="fadeIn second" name="login" placeholder="아이디를 입력해주세요" required>
<input type="text" id="password" class="fadeIn third" name="login" placeholder="비밀번호를 입력해주세요" required>
<input type="submit" class="fadeIn fourth" value="Log In">
</form>
<!-- Remind Passowrd -->
<div id="formFooter">
<a class="underlineHover" href="/auth/join">회원가입</a>
</div>
</div>
</div>
login-js.jsp |
<%@ page contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
<script>
</script>
join.jsp (회원가입 화면) |
<%@ page language="java" session="true" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%>
<%@ include file="/WEB-INF/template/constants.jsp"%>
<link href="//maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css" rel="stylesheet" id="bootstrap-css">
<script src="//maxcdn.bootstrapcdn.com/bootstrap/4.0.0/js/bootstrap.min.js"></script>
<script src="//cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.min.js"></script>
<!------ Include the above in your HEAD tag ---------->
<link rel="stylesheet" type="text/css" href="${ctxPath}/css/loginForm.css"/>
<div class="wrapper">
<div id="formContent">
<!-- Tabs Titles -->
<!-- Icon -->
<div class="fadeIn first">
회원가입
</div>
<!-- Login Form -->
<form>
<input type="text" id="userId" class="fadeIn second" name="login" placeholder="아이디를 입력해주세요." required>
<input type="text" id="password" class="fadeIn third" name="login" placeholder="비밀번호를 입력해주세요." required>
<input type="text" id="name" class="fadeIn third" name="login" placeholder="이름을 입력해주세요.">
<input type="text" id="email" class="fadeIn third" name="login" placeholder="이메일을 입력해주세요.">
<input type="text" id="handPhoneNo" class="fadeIn third" name="login" placeholder="핸드폰번호를 입력해주세요.">
<input type="submit" class="fadeIn fourth" value="Join">
</form>
<!-- Remind Passowrd -->
<div id="formFooter">
<a class="underlineHover" href="/auth/login">뒤로가기</a>
</div>
</div>
</div>
join-js.jsp |
<%@ page contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
<script>
</script>
resources 밑에 static 폴더 생성후 그안에 css 폴더 생성 |
-> css폴더안에 loginForm.css 생성
loginForm.css |
/* BASIC */
html {
background-color: #56baed;
}
body {
font-family: "Poppins", sans-serif;
height: 100vh;
}
a {
color: #92badd;
display:inline-block;
text-decoration: none;
font-weight: 400;
}
h2 {
text-align: center;
font-size: 16px;
font-weight: 600;
text-transform: uppercase;
display:inline-block;
margin: 40px 8px 10px 8px;
color: #cccccc;
}
/* STRUCTURE */
.wrapper {
display: flex;
align-items: center;
flex-direction: column;
justify-content: center;
width: 100%;
min-height: 100%;
padding: 20px;
}
#formContent {
-webkit-border-radius: 10px 10px 10px 10px;
border-radius: 10px 10px 10px 10px;
background: #fff;
padding: 30px;
width: 90%;
max-width: 450px;
position: relative;
padding: 0px;
-webkit-box-shadow: 0 30px 60px 0 rgba(0,0,0,0.3);
box-shadow: 0 30px 60px 0 rgba(0,0,0,0.3);
text-align: center;
}
#formFooter {
background-color: #f6f6f6;
border-top: 1px solid #dce8f1;
padding: 25px;
text-align: center;
-webkit-border-radius: 0 0 10px 10px;
border-radius: 0 0 10px 10px;
}
/* TABS */
h2.inactive {
color: #cccccc;
}
h2.active {
color: #0d0d0d;
border-bottom: 2px solid #5fbae9;
}
/* FORM TYPOGRAPHY*/
input[type=button], input[type=submit], input[type=reset] {
background-color: #56baed;
border: none;
color: white;
padding: 15px 80px;
text-align: center;
text-decoration: none;
display: inline-block;
text-transform: uppercase;
font-size: 13px;
-webkit-box-shadow: 0 10px 30px 0 rgba(95,186,233,0.4);
box-shadow: 0 10px 30px 0 rgba(95,186,233,0.4);
-webkit-border-radius: 5px 5px 5px 5px;
border-radius: 5px 5px 5px 5px;
margin: 5px 20px 40px 20px;
-webkit-transition: all 0.3s ease-in-out;
-moz-transition: all 0.3s ease-in-out;
-ms-transition: all 0.3s ease-in-out;
-o-transition: all 0.3s ease-in-out;
transition: all 0.3s ease-in-out;
}
input[type=button]:hover, input[type=submit]:hover, input[type=reset]:hover {
background-color: #39ace7;
}
input[type=button]:active, input[type=submit]:active, input[type=reset]:active {
-moz-transform: scale(0.95);
-webkit-transform: scale(0.95);
-o-transform: scale(0.95);
-ms-transform: scale(0.95);
transform: scale(0.95);
}
input[type=text] {
background-color: #f6f6f6;
border: none;
color: #0d0d0d;
padding: 15px 32px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 5px;
width: 85%;
border: 2px solid #f6f6f6;
-webkit-transition: all 0.5s ease-in-out;
-moz-transition: all 0.5s ease-in-out;
-ms-transition: all 0.5s ease-in-out;
-o-transition: all 0.5s ease-in-out;
transition: all 0.5s ease-in-out;
-webkit-border-radius: 5px 5px 5px 5px;
border-radius: 5px 5px 5px 5px;
}
input[type=text]:focus {
background-color: #fff;
border-bottom: 2px solid #5fbae9;
}
input[type=text]:placeholder {
color: #cccccc;
}
/* ANIMATIONS */
/* Simple CSS3 Fade-in-down Animation */
.fadeInDown {
-webkit-animation-name: fadeInDown;
animation-name: fadeInDown;
-webkit-animation-duration: 1s;
animation-duration: 1s;
-webkit-animation-fill-mode: both;
animation-fill-mode: both;
}
@-webkit-keyframes fadeInDown {
0% {
opacity: 0;
-webkit-transform: translate3d(0, -100%, 0);
transform: translate3d(0, -100%, 0);
}
100% {
opacity: 1;
-webkit-transform: none;
transform: none;
}
}
@keyframes fadeInDown {
0% {
opacity: 0;
-webkit-transform: translate3d(0, -100%, 0);
transform: translate3d(0, -100%, 0);
}
100% {
opacity: 1;
-webkit-transform: none;
transform: none;
}
}
/* Simple CSS3 Fade-in Animation */
@-webkit-keyframes fadeIn { from { opacity:0; } to { opacity:1; } }
@-moz-keyframes fadeIn { from { opacity:0; } to { opacity:1; } }
@keyframes fadeIn { from { opacity:0; } to { opacity:1; } }
.fadeIn {
opacity:0;
-webkit-animation:fadeIn ease-in 1;
-moz-animation:fadeIn ease-in 1;
animation:fadeIn ease-in 1;
-webkit-animation-fill-mode:forwards;
-moz-animation-fill-mode:forwards;
animation-fill-mode:forwards;
-webkit-animation-duration:1s;
-moz-animation-duration:1s;
animation-duration:1s;
}
.fadeIn.first {
-webkit-animation-delay: 0.4s;
-moz-animation-delay: 0.4s;
animation-delay: 0.4s;
}
.fadeIn.second {
-webkit-animation-delay: 0.6s;
-moz-animation-delay: 0.6s;
animation-delay: 0.6s;
}
.fadeIn.third {
-webkit-animation-delay: 0.8s;
-moz-animation-delay: 0.8s;
animation-delay: 0.8s;
}
.fadeIn.fourth {
-webkit-animation-delay: 1s;
-moz-animation-delay: 1s;
animation-delay: 1s;
}
/* Simple CSS3 Fade-in Animation */
.underlineHover:after {
display: block;
left: 0;
bottom: -10px;
width: 0;
height: 2px;
background-color: #56baed;
content: "";
transition: width 0.2s;
}
.underlineHover:hover {
color: #0d0d0d;
}
.underlineHover:hover:after{
width: 100%;
}
/* OTHERS */
*:focus {
outline: none;
}
#icon {
width:60%;
}
java common 패키지안에 Url.java 만들기 |
package com.board.common;
/* api url 정의 */
public final class Url {
public static final String TILES_ROOT = "/tiles/view";
public static final String TILES_AJAX = "/tiles/ajax";
/* 로그인 */
public static final class AUTH {
/* 로그인 url */
public static final String LOGIN = "/auth/login";
/* 로그인 jsp */
public static final String LOGIN_JSP = TILES_ROOT + "/auth/login";
/* 회원가입 url */
public static final String JOIN = "/auth/join";
/* 회원가입 jsp */
public static final String JOIN_JSP = TILES_ROOT + "/auth/join";
}
}
controller 패키지 밑에 로그인 컨트롤러 생성 |
LoginController.java |
package com.board.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import com.board.common.Url;
@Controller
public class LoginController {
@GetMapping(value= {Url.AUTH.LOGIN})
public String login() {
return Url.AUTH.LOGIN_JSP;
}
@GetMapping(Url.AUTH.JOIN)
public String join() {
return Url.AUTH.JOIN_JSP;
}
}
로그인화면 |
회원가입화면 |
다음포스팅에서는 Spring Securirty를 사용하여 로그인 구현관련 개발을 해보겠습니다.
감사합니다.
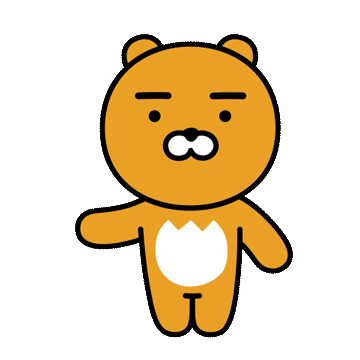
'스프링' 카테고리의 다른 글
[Spring Boot] 4. 게시판 CRUD, 페이징처리 (Gradle+Mybatis+멀티프로젝트+MYSQL+STS) (0) | 2021.08.07 |
---|---|
[Spring Boot] 3. lombok 설정 + 로그인 + 회원가입 개발 (Gradle+Mybatis+멀티프로젝트+MYSQL+STS) (0) | 2021.08.05 |
[Spring Boot] 1. 멀티프로젝트 세팅 (Gradle+Mybatis+멀티프로젝트+MYSQL+STS) (4) | 2021.08.01 |
[Spring] Requset 메소드 사용하기 (0) | 2021.07.05 |
[Spring] 개발환경에서 http 로그 자세히 보기 (0) | 2021.07.05 |