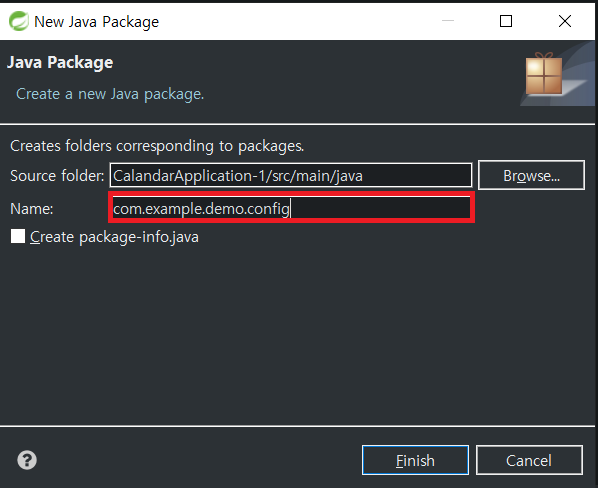
1. com.example.demo.config 패키지 생성
2. 해당 패키지안에 TilesConfig.java 클래스 파일 생성
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.view.UrlBasedViewResolver;
import org.springframework.web.servlet.view.tiles3.TilesConfigurer;
import org.springframework.web.servlet.view.tiles3.TilesView;
@Configuration
public class TilesConfig {
@Bean
public TilesConfigurer tilesConfigurer(){
final TilesConfigurer configurer = new TilesConfigurer();
configurer.setDefinitions(new String[] {"/WEB-INF/tiles.xml"});
configurer.setCheckRefresh(true);
return configurer;
}
@Bean
public UrlBasedViewResolver tilesViewResolver(){
UrlBasedViewResolver resolver = new UrlBasedViewResolver();
resolver.setViewClass(TilesView.class);
return resolver;
}
}
3. WEB-INF 폴더 안에 tiles.xml 생성
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE tiles-definitions PUBLIC "-//Apache Software Foundation//DTD Tiles Configuration 3.0//EN" "http://tiles.apache.org/dtds/tiles-config_3_0.dtd">
<tiles-definitions>
<!-- 화면 레이아웃 템플릿 정의 -->
<definition name="tiles/default/template" template="/WEB-INF/template/template.jsp">
<put-attribute name="meta" value="/WEB-INF/template/common/meta.jsp"/>
<put-attribute name="styles" value="/WEB-INF/template/common/styles.jsp"/>
<put-attribute name="scripts" value="/WEB-INF/template/common/scripts.jsp"/>
<put-attribute name="header" value="/WEB-INF/template/common/header.jsp"/>
</definition>
<!-- Depth(4) : / A / B / C / D / E.jsp -->
<definition name="/tiles/view/*/*/*/*/*" extends="tiles/default/template">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}/{3}/{4}/{5}.jsp"/>
<put-attribute name="contents-js" value="/WEB-INF/views/{1}/{2}/{3}/{4}/{5}-js.jsp"/>
</definition>
<!-- Depth(3) : / A / B / C / D.jsp -->
<definition name="/tiles/view/*/*/*/*" extends="tiles/default/template">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}/{3}/{4}.jsp"/>
<put-attribute name="contents-js" value="/WEB-INF/views/{1}/{2}/{3}/{4}-js.jsp"/>
</definition>
<!-- Depth(2) : / A / B / C.jsp -->
<definition name="/tiles/view/*/*/*" extends="tiles/default/template">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}/{3}.jsp"/>
<put-attribute name="contents-js" value="/WEB-INF/views/{1}/{2}/{3}-js.jsp"/>
</definition>
<!-- Depth(1) : / A / B.jsp -->
<definition name="/tiles/view/*/*" extends="tiles/default/template">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}.jsp"/>
<put-attribute name="contents-js" value="/WEB-INF/views/{1}/{2}-js.jsp"/>
</definition>
<!-- Depth(0) : / A.jsp -->
<definition name="/tiles/view/*" extends="tiles/default/template">
<put-attribute name="contents" value="/WEB-INF/views/{1}.jsp"/>
<put-attribute name="contents-js" value="/WEB-INF/views/{1}-js.jsp"/>
</definition>
<!-- 화면 영역 분할 등에서 사용함 -->
<!-- 관리자 화면 레이아웃 템플릿 정의 -->
<definition name="tiles/default/template-simple" template="/WEB-INF/template/template-simple.jsp">
</definition>
<!-- Depth(4) : / A / B / C / D / E.jsp -->
<definition name="/tiles/ajax/*/*/*/*/*" extends="tiles/default/template-simple">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}/{3}/{4}/{5}.jsp"/>
</definition>
<!-- Depth(3) : / A / B / C / D.jsp -->
<definition name="/tiles/ajax/*/*/*/*" extends="tiles/default/template-simple">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}/{3}/{4}.jsp"/>
</definition>
<!-- Depth(2) : / A / B / C.jsp -->
<definition name="/tiles/ajax/*/*/*" extends="tiles/default/template-simple">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}/{3}.jsp"/>
</definition>
<!-- Depth(1) : / A / B.jsp -->
<definition name="/tiles/ajax/*/*" extends="tiles/default/template-simple">
<put-attribute name="contents" value="/WEB-INF/views/{1}/{2}.jsp"/>
</definition>
<!-- Depth(0) : / A.jsp -->
<definition name="/tiles/ajax/*" extends="tiles/default/template-simple">
<put-attribute name="contents" value="/WEB-INF/views/{1}.jsp"/>
</definition>
<!-- 에러 페이지 레이아웃 -->
<definition name="/tiles/error/template" template="/WEB-INF/template/error/error-template.jsp"/>
<definition name="error.*" extends="tiles/default/template">
<put-attribute name="contents" value="/WEB-INF/views/error/{1}.jsp"/>
</definition>
</tiles-definitions>
4. WEB-INF 폴더안에 template 폴더 생성
5. WEB-INF/template 폴더 안에 template.jsp 파일 생성
<%@ page contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
<%@ taglib prefix="tiles" uri="http://tiles.apache.org/tags-tiles" %>
<!DOCTYPE html>
<html lang="ko">
<head>
<tiles:insertAttribute name="meta"/>
<tiles:insertAttribute name="styles"/>
<tiles:insertAttribute name="scripts"/>
<tiles:insertAttribute name="contents-js" flush="true"/>
</head>
<body>
<!-- wrap -->
<div id="wrap">
<tiles:insertAttribute name="header"/>
<div id="c-mask" class="c-mask"></div><!-- /c-mask -->
<div id="container"><tiles:insertAttribute name="contents"/></div>
</div>
</body>
</html>
6. WEB-INF/template 폴더 안에 template-simple.jsp 파일 생성
<%@ page contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
<%@ taglib prefix="tiles" uri="http://tiles.apache.org/tags-tiles" %>
<tiles:insertAttribute name="contents"/>
* template을 template.jsp, template-simple.jsp로 나누는 이유는 나중에 ajax 통신을 하면서 jsp 파일을 가져올경우
template.jsp 같은경우 안쪽에 meta.jsp, style.jsp, script.jsp, header.jsp를 가져오기 때문에
해당영역만 가져오기위해서 template-simple.jsp를 setting 하였습니다.
7. WEB-INF/template/common 폴더생성
8. WEB-INF/template/common 폴더에 header.jsp 생성
<%@ page contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
<%@ include file="/WEB-INF/template/constants.jsp"%>
9. WEB-INF/template/common 폴더에 meta.jsp 생성
<%@ page contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
<%@ include file="/WEB-INF/prelude.jspf" %>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, minimum-scale=1.0, user-scalable=no, target-densitydpi=device-dpi">
<title>test</title>
<!--
<!-- favicon -->
<link rel="apple-touch-icon" sizes="57x57" href="/favicon/apple-icon-57x57.png">
<link rel="apple-touch-icon" sizes="60x60" href="/favicon/apple-icon-60x60.png">
<link rel="apple-touch-icon" sizes="72x72" href="/favicon/apple-icon-72x72.png">
<link rel="apple-touch-icon" sizes="76x76" href="/favicon/apple-icon-76x76.png">
<link rel="apple-touch-icon" sizes="114x114" href="/favicon/apple-icon-114x114.png">
<link rel="apple-touch-icon" sizes="120x120" href="/favicon/apple-icon-120x120.png">
<link rel="apple-touch-icon" sizes="144x144" href="/favicon/apple-icon-144x144.png">
<link rel="apple-touch-icon" sizes="152x152" href="/favicon/apple-icon-152x152.png">
<link rel="apple-touch-icon" sizes="180x180" href="/favicon/apple-icon-180x180.png">
<link rel="icon" type="image/png" sizes="192x192" href="/favicon/android-icon-192x192.png">
<link rel="icon" type="image/png" sizes="32x32" href="/favicon/favicon-32x32.png">
<link rel="icon" type="image/png" sizes="96x96" href="/favicon/favicon-96x96.png">
<link rel="icon" type="image/png" sizes="16x16" href="/favicon/favicon-16x16.png">
<link rel="manifest" href="/favicon/manifest.json">
<meta name="msapplication-TileColor" content="#ffffff">
<meta name="msapplication-TileImage" content="/favicon/ms-icon-144x144.png">
<meta name="theme-color" content="#ffffff">
<meta name="author" content="renault">
<meta name="robots" content="noindex" />
10. WEB-INF/template/common 폴더에 pageHead.jsp 생성
<%@ page contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
<%@ include file="/WEB-INF/template/constants.jsp"%>
<div class="head">
<div class="back">
<div class="lBtn">
<input type="button" value="btn gnb" id="c-button--push-left" class="c-button btnGnbMobile" />
</div>
<h2><strong>${currentMenu.name}</strong></h2>
<div class="rBtn MrBtn">
<!-- <div> -->
<%-- <span><input type="button" value="HOME" class="btnHome" onclick="location.href='${ct:url('MAIN.INDEX')}' " /></span><!-- otp기능 생성하면서 수정 --> --%>
<%-- <span><input type="button" value="HOME" class="btnHome" onclick="location.href='${ct:url('CONTENTS.LOGIN')}' " /></span> --%>
<%-- <span><input type="button" value="로그아웃" class="btnLogout" onclick="location.href='${ct:url('AUTH.LOGOUT')}' "/></span> --%>
<!-- <span><input type="button" value="사이트 바로가기" class="btnSite" /></span> -->
<!-- </div> -->
</div>
</div>
</div>
11. WEB-INF/template/common 폴더에 scripts.jsp 생성
<%@ page contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
<!-- js -->
<script type="text/javascript" src="${pageContext.request.contextPath}/js/jquery-3.3.1.min.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath}/js/jquery-migrate-1.4.1.min.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath}/js/jquery.form.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath}/js/jquery-ui.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath}/js/duDatepicker.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath}/js/mdtimepicker.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath}/js/swiper.min.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath}/js/jquery.form.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath}/js/jquery.query-object.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath}/js/basic.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath}/js/formstone/core.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath}/js/formstone/upload.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath}/js/alertify.min.js"></script>
<script type="text/javascript" src="${pageContext.request.contextPath}/js/commonFunc.js"></script>
<script>
var CTX_PATH = "${pageContext.request.contextPath}";
</script>
12. WEB-INF/template/common 폴더에 styles.jsp 생성
<%@ page contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
<!-- css -->
<link rel="stylesheet" type="text/css" href="${pageContext.request.contextPath}/css/jquery-ui.css">
<link rel="stylesheet" type="text/css" href="${pageContext.request.contextPath}/css/common.css" />
<link rel="stylesheet" type="text/css" href="${pageContext.request.contextPath}/css/duDatepicker.css">
<link rel="stylesheet" type="text/css" href="${pageContext.request.contextPath}/css/mdtimepicker.css">
<link rel="stylesheet" type="text/css" href="${pageContext.request.contextPath}/css/swiper.min.css">
<link rel="stylesheet" type="text/css" href="${pageContext.request.contextPath}/css/upload.css">
<link rel="stylesheet" type="text/css" href="${pageContext.request.contextPath}/css/alertify.min.css"/>
<link rel="stylesheet" type="text/css" href="${pageContext.request.contextPath}/css/themes/default.min.css">
13. WEB-INF/template/error 폴더 생성
14. WEB-INF/template/error 폴더에 error-template.jsp 생성
<%@ page contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="content-type" content="text/html;charset=UTF-8" />
<meta charset="utf-8" />
<title>Pages - Admin Dashboard UI Kit - Blank Page</title>
<meta name="viewport" content="width=device-width, initial-scale=1.0, maximum-scale=1.0, user-scalable=no, shrink-to-fit=no" />
<link rel="apple-touch-icon" href="pages/ico/60.png">
<link rel="apple-touch-icon" sizes="76x76" href="pages/ico/76.png">
<link rel="apple-touch-icon" sizes="120x120" href="pages/ico/120.png">
<link rel="apple-touch-icon" sizes="152x152" href="pages/ico/152.png">
<link rel="icon" type="image/x-icon" href="favicon.ico" />
<meta name="apple-mobile-web-app-capable" content="yes">
<meta name="apple-touch-fullscreen" content="yes">
<meta name="apple-mobile-web-app-status-bar-style" content="default">
<meta content="" name="description" />
<meta content="" name="author" />
<link href="assets/plugins/pace/pace-theme-flash.css" rel="stylesheet" type="text/css" />
<link href="assets/plugins/bootstrap/css/bootstrap.min.css" rel="stylesheet" type="text/css" />
<link href="assets/plugins/font-awesome/css/font-awesome.css" rel="stylesheet" type="text/css" />
<link href="assets/plugins/jquery-scrollbar/jquery.scrollbar.css" rel="stylesheet" type="text/css" media="screen" />
<link href="assets/plugins/select2/css/select2.min.css" rel="stylesheet" type="text/css" media="screen" />
<link href="assets/plugins/switchery/css/switchery.min.css" rel="stylesheet" type="text/css" media="screen" />
<link href="pages/css/pages-icons.css" rel="stylesheet" type="text/css">
<link class="main-stylesheet" href="pages/css/pages.css" rel="stylesheet" type="text/css" />
<script type="text/javascript">
window.onload = function()
{
// fix for windows 8
if (navigator.appVersion.indexOf("Windows NT 6.2") != -1)
document.head.innerHTML += '<link rel="stylesheet" type="text/css" href="pages/css/windows.chrome.fix.css" />'
}
</script>
</head>
<body class="fixed-header error-page">
<!-- BEGIN ERROR BODY -->
<!-- END ERROR BODY -->
<!-- BEGIN VENDOR JS -->
<script src="assets/plugins/pace/pace.min.js" type="text/javascript"></script>
<script src="assets/plugins/jquery/jquery-1.11.1.min.js" type="text/javascript"></script>
<script src="assets/plugins/modernizr.custom.js" type="text/javascript"></script>
<script src="assets/plugins/jquery-ui/jquery-ui.min.js" type="text/javascript"></script>
<script src="assets/plugins/tether/js/tether.min.js" type="text/javascript"></script>
<script src="assets/plugins/bootstrap/js/bootstrap.min.js" type="text/javascript"></script>
<script src="assets/plugins/jquery/jquery-easy.js" type="text/javascript"></script>
<script src="assets/plugins/jquery-unveil/jquery.unveil.min.js" type="text/javascript"></script>
<script src="assets/plugins/jquery-ios-list/jquery.ioslist.min.js" type="text/javascript"></script>
<script src="assets/plugins/jquery-actual/jquery.actual.min.js"></script>
<script src="assets/plugins/jquery-scrollbar/jquery.scrollbar.min.js"></script>
<script type="text/javascript" src="assets/plugins/select2/js/select2.full.min.js"></script>
<script type="text/javascript" src="assets/plugins/classie/classie.js"></script>
<script src="assets/plugins/switchery/js/switchery.min.js" type="text/javascript"></script>
<!-- END VENDOR JS -->
<script src="pages/js/pages.min.js"></script>
</body>
</html>
15. WEB-INF/template 폴더에 constant.jsp 파일 생성
<%@ page language="java" session="true" contentType="text/html; charset=utf-8" pageEncoding="utf-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core" %>
<%@ taglib prefix="fmt" uri="http://java.sun.com/jsp/jstl/fmt" %>
<%@ taglib prefix="fn" uri="http://java.sun.com/jsp/jstl/functions" %>
<%@ taglib prefix="spring" uri="http://www.springframework.org/tags" %>
<%-- <%@ taglib prefix="sec" uri="http://www.springframework.org/security/tags" %> --%>
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form" %>
<%-- <%@ taglib prefix="ct" uri="/WEB-INF/tlds/common-taglibs.tld" %> --%>
<c:set var="ctxPath" value="${pageContext.request.contextPath eq '/' ? '' : pageContext.request.contextPath}" />
<c:set var="remoteURI" value="${ctxPath}${requestScope['javax.servlet.forward.servlet_path']}" />
16. WEB-INF 폴더안에 prelude.jspf 파일 생성
<%@ page contentType="text/html; charset=utf-8" pageEncoding="utf-8" %>
17. mainController 수정
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class MainController {
@RequestMapping("/")
public String main() {
return "/tiles/view/main";
}
}
18. build.gradle 수정
plugins {
id 'org.springframework.boot' version '2.1.8.RELEASE'
id 'io.spring.dependency-management' version '1.0.8.RELEASE'
id 'java'
id 'war'
}
group = 'com.example'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '1.8'
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-web'
providedRuntime 'org.springframework.boot:spring-boot-starter-tomcat'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
//tomcat
compile 'org.apache.tomcat.embed:tomcat-embed-jasper'
//jstl
compile 'javax.servlet:jstl:1.2'
//tiles
compile group: 'org.apache.tiles', name: 'tiles-jsp', version: '3.0.7'
}
jstl, tiles 부분 추가
디렉터리 구조
//결과화면
tiles setting이 끝난걸 확인할수 있습니다.
'스프링' 카테고리의 다른 글
[4] Spring boot Mybatis + Mysql + gradle 설정 (0) | 2019.09.28 |
---|---|
[3] Spring boot 롬복 설치 (0) | 2019.09.28 |
[1] Spring Boot 웹프로젝트 만들기 (0) | 2019.09.28 |
[Spring] 파일업로드 (0) | 2019.07.19 |
[Spring] 저장된 파일 이미지 보여주기 (0) | 2019.07.19 |