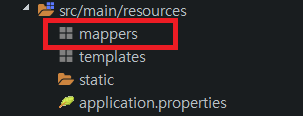
1. application.properties 수정
spring.mvc.view.prefix=/WEB-INF/views/
spring.mvc.view.suffix=.jsp
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/calandar_db
spring.datasource.username=root
spring.datasource.password=1234
2. build.gradle 수정
plugins {
id 'org.springframework.boot' version '2.1.8.RELEASE'
id 'io.spring.dependency-management' version '1.0.8.RELEASE'
id 'java'
id 'war'
}
group = 'com.example'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '1.8'
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-web'
providedRuntime 'org.springframework.boot:spring-boot-starter-tomcat'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
//tomcat
compile 'org.apache.tomcat.embed:tomcat-embed-jasper'
//jstl
compile 'javax.servlet:jstl:1.2'
//tiles
compile group: 'org.apache.tiles', name: 'tiles-jsp', version: '3.0.7'
//lombok
compile "org.projectlombok:lombok:1.16.6"
// MyBatis
compile("org.springframework.boot:spring-boot-starter-jdbc:2.0.1.RELEASE")
compile("mysql:mysql-connector-java:5.1.46")
compile("org.mybatis.spring.boot:mybatis-spring-boot-starter:1.3.1")
compile("org.mybatis:mybatis-spring:1.3.1")
compile("org.mybatis:mybatis:3.4.5")
}
//mybatis 부분을 추가한다.
3. app.java 수정
import javax.sql.DataSource;
import org.apache.ibatis.session.SqlSessionFactory;
import org.mybatis.spring.SqlSessionFactoryBean;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.core.io.Resource;
import org.springframework.core.io.support.PathMatchingResourcePatternResolver;
@SpringBootApplication
@MapperScan(value={"member.service.mapper"})
public class CalandarApplication1Application {
public static void main(String[] args) {
SpringApplication.run(CalandarApplication1Application.class, args);
}
@Bean
public SqlSessionFactory sqlSessionFactory(DataSource dataSource) throws Exception{
SqlSessionFactoryBean sessionFactory = new SqlSessionFactoryBean();
sessionFactory.setDataSource(dataSource);
Resource[] res = new PathMatchingResourcePatternResolver().getResources("classpath:mappers/*Mapper.xml");
sessionFactory.setMapperLocations(res);
return sessionFactory.getObject();
}
}
4. src./main/resources 폴더안에 mappers 패키지를 만든다.
5. controller, service, serviceImpl, mapper , mapper.xml 세팅
CalandarVO
import lombok.Getter;
import lombok.Setter;
/*
* 캘린더 VO
*/
@Getter
@Setter
public class CalandarVO {
private String calandarIdx; //캘린더 idx
private String title; //타이틀
private String content; //컨텐츠
private String regDate; //등록일
}
MainController
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import com.example.demo.service.CalandarService;
import com.example.demo.vo.CalandarVO;
@Controller
public class MainController {
@Autowired CalandarService calandarService;
@GetMapping("/")
public String main(CalandarVO calandarVO) {
//캘린더 리스트 조회
List<CalandarVO> selectCalandarList = calandarService.selectCalandarList(calandarVO);
System.out.println(selectCalandarList.size());
return "/tiles/view/main";
}
}
CalandarService
import java.util.List;
import com.example.demo.vo.CalandarVO;
/*
* 캘린더 서비스
*/
public interface CalandarService {
//캘린더 리스트 조회
List<CalandarVO> selectCalandarList(CalandarVO calandarVO);
}
CalandarMapper
import java.util.List;
import com.example.demo.vo.CalandarVO;
/*
* 캘린더 Mapper
*/
public interface CalandarMapper {
//캘린더 리스트 조회
List<CalandarVO> selectCalandarList(CalandarVO calandarVO);
}
CalandarServiceImpl
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.example.demo.mapper.CalandarMapper;
import com.example.demo.service.CalandarService;
import com.example.demo.vo.CalandarVO;
@Service("calandarService")
public class CalandarServiceImpl implements CalandarService {
@Autowired CalandarMapper calandarMapper;
/*
* (non-Javadoc)
* @see com.example.demo.service.CalandarService#selectCalandarList(com.example.demo.vo.CalandarVO)
*/
@Override
public List<CalandarVO> selectCalandarList(CalandarVO calandarVO) {
return calandarMapper.selectCalandarList(calandarVO);
}
}
CalandarMapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.demo.mapper.CalandarMapper">
<!-- 캘린더 리스트 조회 -->
<select id="selectCalandarList" parameterType="com.example.demo.vo.CalandarVO" resultType="com.example.demo.vo.CalandarVO">
SELECT
CALANDAR_IDX
,TITLE
,CONTENT
,REG_DATE
FROM
CALANDAR_LIST
</select>
</mapper>
결과화면
WARN으로 코드들이 나오는데 이코드들을 감추려면
application.properties에 ?useSSL-false를 붙이면 깔끔하게 나옵니다.
디렉터리 구조
'스프링' 카테고리의 다른 글
이클립스 패키지 일괄변경 (0) | 2020.10.06 |
---|---|
[Spring] 엑셀 다운로드 (0) | 2019.11.28 |
[3] Spring boot 롬복 설치 (0) | 2019.09.28 |
[2] Spring Boot TilesConfig 설정 (0) | 2019.09.28 |
[1] Spring Boot 웹프로젝트 만들기 (0) | 2019.09.28 |